diff options
author | Eug-VS <eug-vs@keemail.me> | 2020-02-08 15:31:14 +0300 |
---|---|---|
committer | eug-vs <eug-vs@keemail.me> | 2020-04-03 03:06:05 +0300 |
commit | eee96f01e29ddb8b95e9429c4e584fe6e7e7faec (patch) | |
tree | 9db6908c0ce8907ac8db74871ea7ab1d324cef9b | |
parent | 2b076ec2796712a12891afb95b3d65311a04e9cb (diff) | |
download | react-benzin-eee96f01e29ddb8b95e9429c4e584fe6e7e7faec.tar.gz |
feat: implement initial markdown component
-rw-r--r-- | src/index.tsx | 17 | ||||
-rw-r--r-- | src/lib/Markdown/Markdown.tsx | 57 | ||||
-rw-r--r-- | src/lib/Markdown/example.md | 50 | ||||
-rw-r--r-- | src/lib/index.ts | 1 |
4 files changed, 112 insertions, 13 deletions
diff --git a/src/index.tsx b/src/index.tsx index 9d32585..cba71b6 100644 --- a/src/index.tsx +++ b/src/index.tsx @@ -10,6 +10,7 @@ import { ContentSection, SmartList, Button, + Markdown, } from './lib'; import icon from './assets/icon.svg'; @@ -27,6 +28,8 @@ const useStyles = makeStyles(theme => ({ })); +const data = "# Getting started\n## Installation \nGo and install it \n# Development\nMore info on dev"; + const Icon = <img src={icon} width="32px" height="37px" alt="logo"/> const headerContents = { @@ -89,19 +92,7 @@ const App: React.FC = () => { primary </Button> </ContentSection> - <ContentSection sectionName="Content section"> - <p> - Fusce suscipit, wisi nec facilisis facilisis, est dui fermentum leo, quis tempor ligula erat quis odio. Nunc porta vulputate tellus. Nunc rutrum turpis sed pede. Sed bibendum. Aliquam posuere. - </p> - <p> - <Link href="#">Link example</Link> - </p> - </ContentSection> - <ContentSection sectionName="Content section"> - <p> - Lorem ipsum dolor sit amet, consectetuer adipiscing elit. Donec hendrerit tempor tellus. Donec pretium posuere tellus. Proin quam nisl, tincidunt et, mattis eget, convallis nec, purus. Cum sociis natoque penatibus et magnis dis parturient montes, nascetur ridiculus mus. Nulla posuere. Donec vitae dolor. Nullam tristique diam non turpis. Cras placerat accumsan <Link href="#">nulla</Link>. Nullam rutrum. Nam vestibulum accumsan nisl. Pellentesque dapibus suscipit ligula. - </p> - </ContentSection> + <Markdown data={data} /> </div> </Window> <Window type="secondary" name="SmartList preview window"> diff --git a/src/lib/Markdown/Markdown.tsx b/src/lib/Markdown/Markdown.tsx new file mode 100644 index 0000000..a3ffc38 --- /dev/null +++ b/src/lib/Markdown/Markdown.tsx @@ -0,0 +1,57 @@ +import React from 'react'; + +import ContentSection from '../ContentSection/ContentSection'; + + +interface PropTypes { + data: string; +} + +interface RawLinesPropType { + rawLines: string[]; + level?: number; +} + +const header = (level: number): string => { + return `^#{${level}} .*$`; +}; + +const Content: React.FC<RawLinesPropType> = ({ rawLines }) => { + const plainText = rawLines.join(); + return <p> {plainText} </p>; +} + +const Level: React.FC<RawLinesPropType> = ({ rawLines, level = 0 }) => { + const name = rawLines[0].slice(level); + const contentSize = rawLines.findIndex(line => line.match(header(level + 1))); + + const rawContent = (contentSize > 0) ? rawLines.slice(1, contentSize) : rawLines.slice(1); + const rawChildren = rawLines.slice(contentSize); + + const childrenLineGroups = rawChildren.reduce((acc: any[], cur: string) => { + if (cur.match(header(level + 1))) acc.push([]); + if (acc.length) acc[acc.length - 1].push(cur); + return acc; + }, []); + const children = childrenLineGroups.map(lineGroup => <Level rawLines={lineGroup} level={level + 1}/>) + + return level ? ( + <ContentSection sectionName={name}> + <Content rawLines={rawContent} /> + {children} + </ContentSection> + ) : ( + <> + {children} + </> + ); +} + +const Markdown: React.FC<PropTypes> = ({ data }) => { + const rawLines = data.split('\n'); + return <Level rawLines={rawLines} /> +}; + + +export default Markdown; + diff --git a/src/lib/Markdown/example.md b/src/lib/Markdown/example.md new file mode 100644 index 0000000..abc0910 --- /dev/null +++ b/src/lib/Markdown/example.md @@ -0,0 +1,50 @@ +# Getting started +## Installation +You can easily add **BENZIN** to your project with `npm`: +```bash +$ npm install react-benzin +``` +**BENZIN** works best in kick-starting new projects and allows you to focus on the functionality, while all the beauty will be maintained by our library. + +**TIP:** *Create-React-App with Typescript* is your GO-TO in most of the cases. [Learn more.](https://create-react-app.dev/docs/adding-typescript/) + +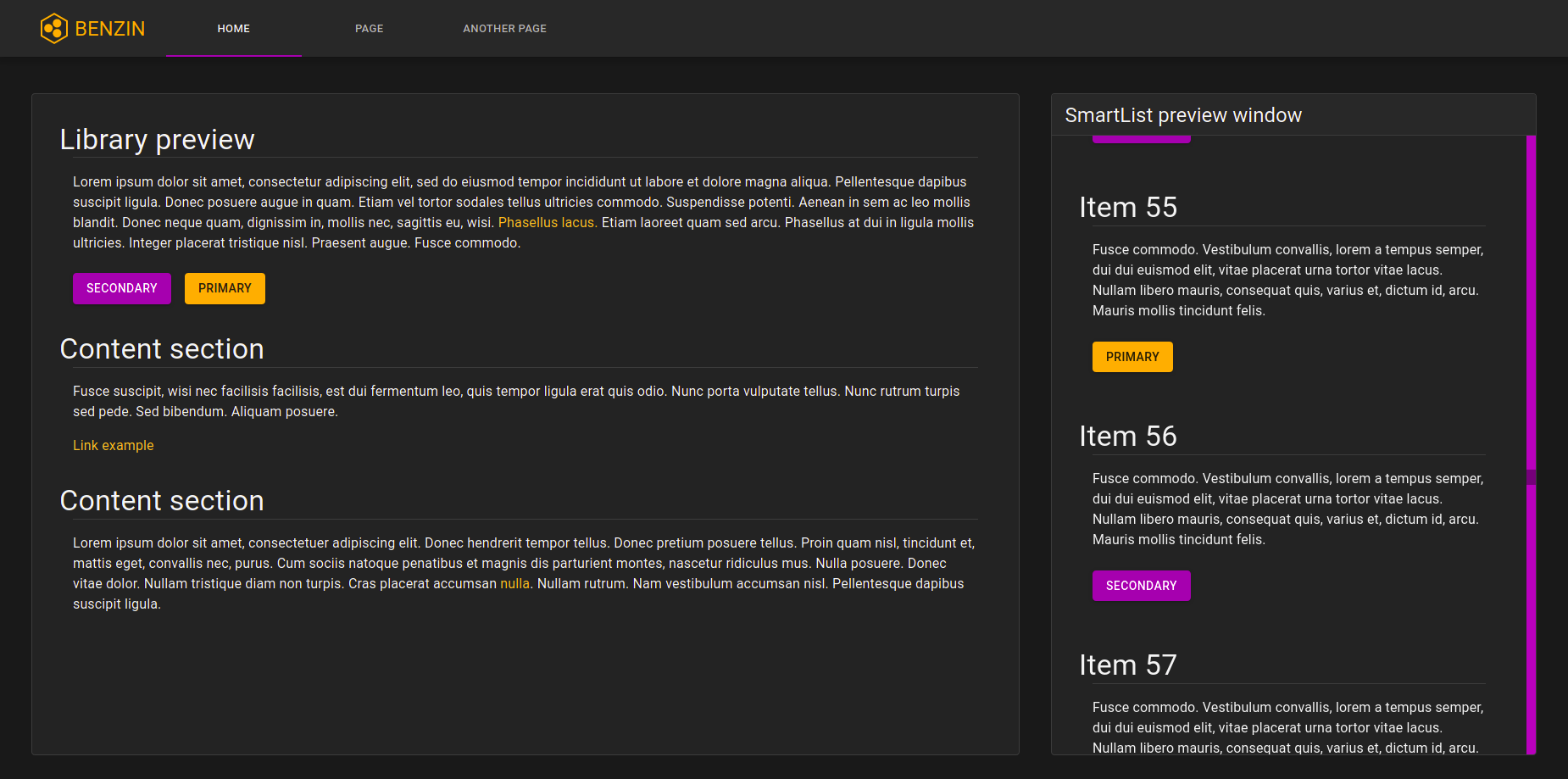 + +You can find a minimal usage example [here](src/index.tsx). + +## Functionality +**BENZIN** provides you with a bunch of cool components that greatly integrate with each other. + +[Explore](src/lib) `src/lib/` folder to see what's available. Documentation is yet to come, but for now you can enjoy type definitons. + +[Chrono-Cube](https://github.com/eug-vs/chrono-cube/) will also be a great example of usage, since it's the actual project which inspired us to create **BENZIN**. + + +# Explore NPM package online +https://www.npmjs.com/package/react-benzin + + +# Development +## Running live demo +To run a live example, clone a repo and execute following commands: +```bash +$ npm i +$ npm start +``` +It's worth noticing that presence of React-App in this repo forces us to split some configurations. For example, we have 2 `Typescript` configs: one for `react-scripts` to run live-demo, and the other one to build *distribution files*. + +## Running tests +```bash +$ npm test +``` +**NOTE**: this command assures that `ESlint` does not throw any warnings and exits with a *non-zero status code* otherwise. That means `CircleCI` tests would fail *even if a single warning is present*. Therefore, you should always locally test your changes before publishing them. + +## Building +We've decided to use `Typescript compiler` to transpile our code, since we think `Babel` is a bit of an overkill here. +```bash +$ npm run build +``` +This command will generate `dist/` folder ready for distribution, which you of course can explore. Note that `tsc` creates type definitions (`.d.ts`) for every corresponding `.js` file. It's very useful because consumers also get access to them. + +## Deploying +Deploying to `npm` is fully automated through **CircleCI**: simply tag a commit as a Release and it will do the job. diff --git a/src/lib/index.ts b/src/lib/index.ts index a41dd39..121c908 100644 --- a/src/lib/index.ts +++ b/src/lib/index.ts @@ -4,3 +4,4 @@ export { default as ContentSection } from './ContentSection/ContentSection'; export { default as SmartList } from './SmartList/SmartList'; export { default as BenzinThemeProvider } from './BenzinThemeProvider/BenzinThemeProvider'; export { default as Button } from './Button/Button'; +export { default as Markdown } from './Markdown/Markdown'; |